Create a Discord Login Web Page using Next.js
When it comes to building a Discord authentication page, Next.js can provide a number of benefits. In this article, you'll learn how to do exactly that.


What is Next.js?
Next.js is an open-source web development framework created by Vercel enabling React-based web applications with server-side rendering and generating static websites. You can learn Next.js in the simplest way possible through their official documentation site. They have listed lessons starting from the basics of the web development framework. Start learning Next.js today!
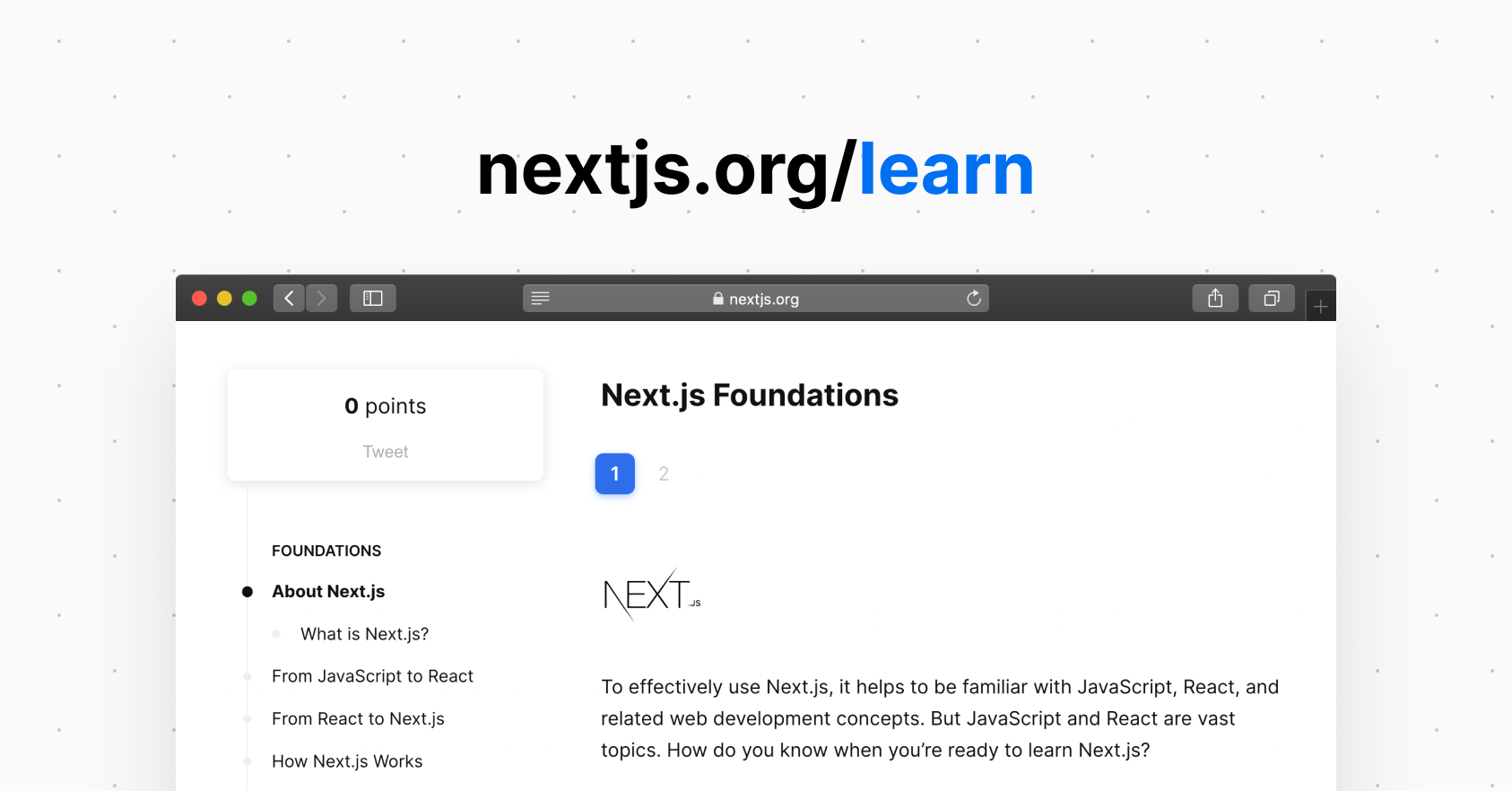
React documentation mentions Next.js among "Recommended Toolchains" advising it to developers as a solution when "Building a server-rendered website with Node.js. Where traditional React apps can only render their content in the client-side browser, Next.js extends this functionality to include applications rendered on the server-side.
Purpose of using Next.js in this guide
When it comes to building a Discord authentication page, Next.js can provide a number of benefits, including:
- Easy setup: Next.js makes it easy to set up a new project and get started with building your application. It also provides a built-in development server, so you can start building your application right away.
- Scalability: Next.js applications can be scaled to handle large amounts of traffic, making it well-suited for building high-performance applications.
- Server-side rendering: Next.js allows developers to easily create server-rendered React applications. This can be useful when building an authentication page, as it allows you to securely handle user credentials on the server, rather than exposing them to the client.
- Easy to use with other library: Next.js also provides an easy way to use other library such as
next-auth
which allow you to easily handle the OAuth flow and manage user sessions, this can help you to implement the Discord authentication page quickly. - Easy Deployment: Next.js can be easily deployed to various hosting providers such as Vercel, Zeit, AWS, and more. These providers offer easy and seamless integration with Next.js, making deployment a breeze.
The next-auth module
In this guide, we are going to achieve a web page allowing us to authorize our Discord accounts by logging in (on the page we created of course) by using the next-auth
module.

next-auth
is a popular library that can be used to easily add authentication to a Next.js application. It provides a simple, flexible API that allows developers to quickly add authentication functionality to their application without having to write a lot of boilerplate code. We will use this module and configure it to work with Discord.
Setting up the Environment and Installing Packages
First, you'll need to make sure you have a Next.js project set up. You can do this by running the following command:
COMMANDLINE
npx create-next-app my-app
You will have your environment ready. It will look like the image below, and you will find all the necessary files needed to make and run the project. You will also find the pages directory consisting of the default index.js
and other page files. We will be creating the main file for this project in this directory.
Next, you'll need to install the next-auth
package, which can be done by running the following command:
COMMANDLINE
npm install next-auth
Once you have next-auth
installed, you'll need to configure it to work with Discord.
next-auth.config.js
file, you will need to configure the next-auth
to work with Discord by following the instructions provided in the next-auth
documentation.
Once you have your next-auth
configuration set up, you can create a login page by creating a new file called login.js
in your pages
directory. This file should import the useSession
hook from next-auth
and use it to check if the user is already logged in. If they are not logged in, you can render a login form that allows them to enter their Discord credentials.
Finally, you'll need to handle the form submission and use the signIn
method provided by next-auth
to log the user in.
Your final code will look like this
import { useSession } from 'next-auth/client'
export default function Login() {
const [session, loading] = useSession()
if (session) {
return <p>You are logged in as {session.user.email}</p>
}
if (loading) {
return <p>Loading...</p>
}
return (
<form onSubmit={handleSubmit}>
<label>
Email:
<input type="email" name="email" required />
</label>
<label>
Password:
<input type="password" name="password" required />
</label>
<button type="submit">Login</button>
</form>
)
async function handleSubmit(event) {
event.preventDefault()
const formData = new FormData(event.currentTarget)
const email = formData.get('email')
const password = formData.get('password')
try {
await signIn({ email, password })
} catch (error) {
console.error(error)
}
}
}
Final Result
- Start the development server by running the command
npm run dev
in your project directory. This will start the development server and make your application available athttp://localhost:3000
. - In your browser, navigate to
http://localhost:3000/login
. You should now see the login form on its first execution.
That's all for today, hope you enjoyed today's article. Make sure to join our official Discord server to discuss further on this topic or suggest new articles!
Like what you're reading?
We do this everyday. Unlock exclusive benefits, 4K wallpapers, and more. Become a member for the price of a coffee.